Welcome to Week 3–4 of your GATE CS/IT journey! If you’ve already laid the foundation with Programming and Data Structures, it’s time to tackle the core of computational thinking—Algorithms.
This blog dives deep into the must-know topics of Algorithms for GATE CS/IT, including Searching, Sorting, Divide & Conquer, Greedy Algorithms, Dynamic Programming, Graph Algorithms, and Backtracking. With structured inputs from Gate AT Zeal, you’ll also get expert tips and targeted strategies to crack this high-weightage section efficiently.
Table of Contents
Week 3: Searching, Sorting & Divide & Conquer
Searching Algorithms
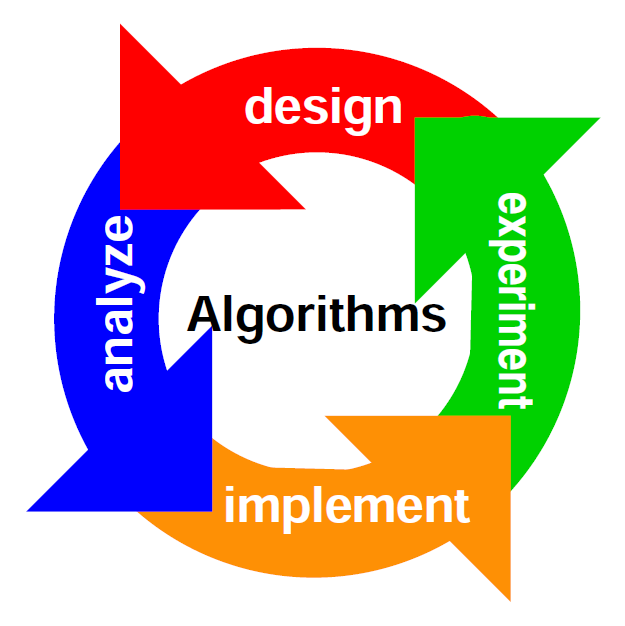
- Topics:
- Linear Search
- Binary Search (Iterative & Recursive)
- Search in Rotated Sorted Arrays
- Why It Matters: Binary Search is the base of many advanced topics (e.g., order statistics)
- Common GATE PYQs: Time complexity of search operations in arrays and trees
- Gate AT Zeal Tip: Practice binary search-based MCQs in quizzes for speed and accuracy.
Sorting Algorithms
- Topics:
- Bubble, Insertion, Selection Sort
- Merge Sort, Quick Sort, Heap Sort
- Counting, Radix, and Bucket Sort (Non-Comparison Based)
- Time Complexity Recap:
Sorting Algorithm | Best Case | Worst Case | Stable? |
---|---|---|---|
Merge Sort | O(n log n) | O(n log n) | Yes |
Quick Sort | O(n log n) | O(n²) | No |
Heap Sort | O(n log n) | O(n log n) | No |
Insertion Sort | O(n) | O(n²) | Yes |
Also Read: How to Avoid Silly Mistakes & Negative Marking in GATE Exam? | GATE 2025
Pro Tip: Focus on internal vs external sorting, and sorting-based MCQs in GATE PYQs.
Divide & Conquer
- Concepts:
- Recurrence Relations (T(n) = T(n/2) + O(n))
- Merge Sort, Binary Search, Quick Sort
- Must-Know: Apply Master Theorem for time complexity analysis.
- GATE Focus Areas: Recursion trees and solving divide-and-conquer recurrences.
Week 4: Greedy, Dynamic Programming, Graphs & Backtracking
Greedy Algorithms
- Topics:
- Activity Selection, Fractional Knapsack
- Huffman Coding, MST using Kruskal’s/Prim’s
- Gate AT Zeal Strategy:
- Learn when greedy fails (compare with DP)
- Solve at least 10 PYQs from previous GATE papers
Dynamic Programming (DP)
- Topics:
- 0/1 Knapsack, Matrix Chain Multiplication
- Longest Common Subsequence (LCS), Edit Distance
- Optimal BST, Coin Change
- Approach:
- Bottom-up vs Top-down (memoization)
- State Transition Diagrams
- Common Mistake: Not identifying overlapping subproblems—practice distinguishing DP vs Greedy!
Zeal Insight: Attend weekly problem-solving labs at Gate AT Zeal for in-depth DP workshops.
Also Read: GATE CSE IMP Topics Subject-wise Breakdown | Gate CSE Important Topics
Graph Algorithms
- Topics:
- DFS, BFS, Topological Sort
- Dijkstra’s, Bellman-Ford, Floyd-Warshall
- Kruskal’s, Prim’s, Disjoint Sets (Union-Find)
- Weightage: Consistently 8–10 marks in GATE
- Concepts to Master:
- Adjacency matrix vs list
- Detecting cycles in directed/undirected graphs
- Shortest paths and MST
Tools: Use visualization tools like VisuAlgo or GeeksforGeeks’ animations for better understanding.
Backtracking
- Topics:
- N-Queens, Subset Sum, Permutations
- Rat in a Maze, Sudoku Solver
- Key Techniques:
- Recursion with constraints
- Pruning and feasibility checks
GATE Angle:
- Focused mainly on implementation-style MSQs/NATs
- Important for advanced algorithm-based questions
Zeal’s Weekly Action Plan: Week 3–4
Week | Topic Areas | Task | Goal |
---|---|---|---|
3 | Searching, Sorting, Divide & Conquer | Study + 30 PYQs + 2 Mock Tests | Concept & speed boost |
4 | DP, Greedy, Graphs, Backtracking | Labs + 40 PYQs + 2 Mock Tests | Master algorithmic thinking |
Tips from Gate AT Zeal Mentors
- Visualize: Sketch recursion trees and graph traversals.
- Trace Code: Dry-run functions manually for DP and Greedy problems.
- Formula Sheets: Maintain quick formula notes for recurrence relations.
- Mock Analysis: Don’t just take tests—analyze what and why you got wrong.
Also Read: 14 Hidden Gems: Must-Know CSMA/CD Questions for GATE CS/IT | Computer Network Questions
Conclusion
Algorithms are not just another subject—they’re the heart of GATE CS/IT. When mastered, they can help you solve a broad range of problems faster and smarter. With this Week 3–4 plan, expert guidance from Gate AT Zeal, and consistent practice, you’re well on your way to scoring big in the Algorithms section.
So sharpen your logic, trust the process, and keep coding!
FAQs
How much weightage do algorithms carry in the GATE CS/IT exam?
Algorithms typically contribute around 10–12 marks in the GATE CS/IT paper, making it one of the most critical topics to focus on.
What are the most frequently asked algorithm topics in GATE?
Dynamic Programming, Greedy Algorithms, Sorting Techniques, and Graph Algorithms like Dijkstra and Kruskal are common in GATE papers.
How can I differentiate between when to use Greedy vs Dynamic Programming?
Greedy is used when a locally optimal choice leads to a globally optimal solution. Use DP when subproblems overlap and greedy fails to produce the best result.
What’s the best way to practice graph algorithms for GATE?
Start by understanding BFS, DFS, and shortest path algorithms. Then practice problems from previous GATE papers and use visualization tools like VisuAlgo.
Do I need to memorize sorting algorithm complexities for GATE?
Yes. You should know best, average, and worst-case complexities of all standard sorting algorithms, especially Merge Sort, Quick Sort, and Heap Sort.
Is backtracking important for GATE CS/IT?
Yes, though it appears less frequently than other topics, backtracking is tested through conceptual and coding-based NAT questions.
Need help with problem-solving strategies? Book a doubt-clearing session with Gate AT Zeal today!